Java Http请求工具类 基于最新版 HttpClient5 Fluent home 编辑时间 2023/04/13 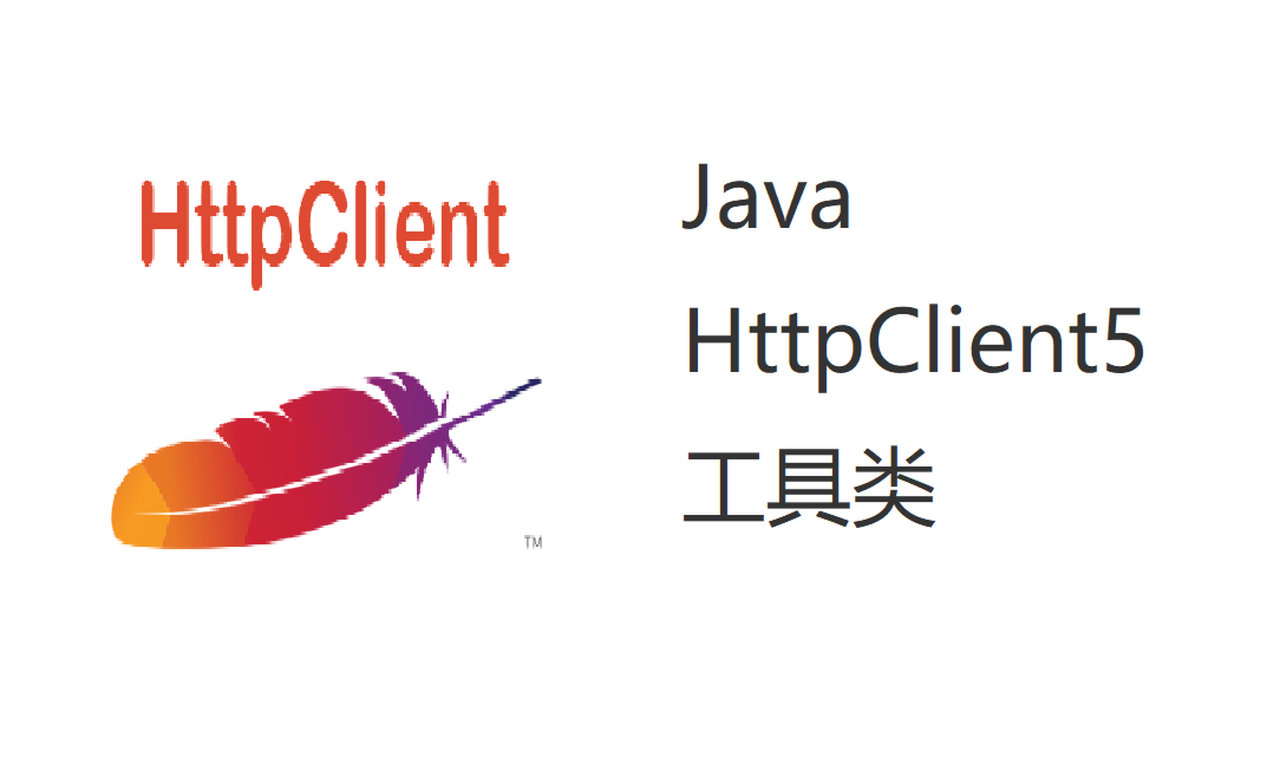 ## 前言 Java项目Http请求工具类 基于Apache HttpClient依赖最新版 ## 折腾 最基本的依赖是 其中最核心的是`httpclient5` 另一个`httpclient5-fluent`是简化代码用的 ```xml <dependency> <groupId>org.apache.httpcomponents.client5</groupId> <artifactId>httpclient5</artifactId> <version>5.2.1</version> </dependency> <dependency> <groupId>org.apache.httpcomponents.client5</groupId> <artifactId>httpclient5-fluent</artifactId> <version>5.2.1</version> </dependency> ``` <br> **先看一个错误示范** 这个报错是因为只引入第二个依赖 `httpclient5-fluent` 第一个核心没引入,就会出现反射错误 ```java Exception in thread "main" java.lang.NoClassDefFoundError: org/apache/hc/client5/http/config/ConnectionConfig at org.apache.hc.client5.http.fluent.Executor.<clinit>(Executor.java:68) at org.apache.hc.client5.http.fluent.Request.execute(Request.java:205) at com.joinzs.auction.utils.HttpClientUtils.httpGet(HttpClientUtils.java:82) at com.joinzs.auction.utils.HttpClientUtils.main(HttpClientUtils.java:105) Caused by: java.lang.ClassNotFoundException: org.apache.hc.client5.http.config.ConnectionConfig at java.net.URLClassLoader.findClass(URLClassLoader.java:387) at java.lang.ClassLoader.loadClass(ClassLoader.java:418) at sun.misc.Launcher$AppClassLoader.loadClass(Launcher.java:352) at java.lang.ClassLoader.loadClass(ClassLoader.java:351) ... 4 more ``` <br> **我的工具类里还用到一个依赖 fastjson** ```xml <dependency> <groupId>com.alibaba.fastjson2</groupId> <artifactId>fastjson2</artifactId> <version>2.0.27</version> </dependency> ``` 工具类代码 ```java import com.alibaba.fastjson2.JSON; import com.alibaba.fastjson2.JSONObject; import org.apache.commons.lang3.StringUtils; import org.apache.hc.client5.http.fluent.Request; import org.apache.hc.core5.http.ContentType; import org.apache.hc.core5.http.io.entity.StringEntity; import java.io.IOException; import java.util.Map; /** * HTTP 请求工具类 * * @author zzzmh * @email admin@zzzmh.cn * @date 2023-4-10 11:25:35 * <p> * 参考 * https://hc.apache.org/httpcomponents-client-5.2.x/quickstart.html * https://blog.csdn.net/hebiwen95/article/details/126405108 * <p> */ public class HttpClientUtils { public static JSONObject doPost(String url, JSONObject header, JSONObject params) { return doPost(url, JSON.toJSONString(header), JSON.toJSONString(params)); } public static JSONObject doPost(String url, JSONObject params) { return doPost(url, JSON.toJSONString(params)); } public static JSONObject doGet(String url) { return doGet(url, null); } public static JSONObject doPost(String url, String header, String params) { return JSONObject.parseObject(httpPost(url, header, params)); } public static JSONObject doPost(String url, String params) { return doPost(url, null, params); } public static JSONObject doGet(String url, String header) { return JSONObject.parseObject(httpGet(url, header)); } /** * 发送 http post 请求 基本款 * * @param url 请求 url * @param header 头部参数 JSON格式的String * @param params 请求参数 JSON格式的String * @return 返回数据 JSON格式的String */ public static String httpPost(String url, String header, String params) { String result = null; try { Request request = Request.post(url); addHeader(request, header); addParams(request, params); result = request.execute().returnContent().asString(); } catch (IOException e) { e.printStackTrace(); } return result; } /** * 发送 http get 请求 基本款 * * @param url 请求 url 自己拼参数 * @param header 头部参数 JSON格式的String * @return 返回数据 JSON格式的String */ public static String httpGet(String url, String header) { String result = null; try { Request request = Request.get(url); addHeader(request, header); result = request.execute().returnContent().asString(); } catch (IOException e) { e.printStackTrace(); } return result; } public static void addHeader(Request request, String header) { if (StringUtils.isNotBlank(header)) { JSONObject headers = JSONObject.parseObject(header); for (String key : headers.keySet()) { request.addHeader(key, headers.getString(key)); } } } public static void addParams(Request request, String json) { if (StringUtils.isNotBlank(json)) { request.body(new StringEntity(json, ContentType.APPLICATION_JSON)); } } } ``` <br> 使用方法 **非JSON请求** ```java public static void main(String[] args) { String get = httpGet("http://www.baidu.com", null); } ``` **JSON请求** ```java public static void main(String[] args) { JSONObject object = doPost("https://api.xxx.com", JSONObject.of("参数a", "a", "参数b", "b")); } ``` ### END 参考 https://hc.apache.org/httpcomponents-client-5.2.x/quickstart.html https://blog.csdn.net/hebiwen95/article/details/126405108 送人玫瑰,手留余香 赞赏 Wechat Pay Alipay 微信小程序 Java服务端 登录相关代码 工具类 2023最新版 关于利用 Github 实现 Jsdelivr 免费 CDN 教程